The steps to integrate the PhonePe Payment Gateway with flutter platform. We provide a plugin/bridge to integrate our SDK.
The minimum supported versions
- Latest Flutter SDK Version: 2.0.2
We can implement with the below 2 steps:
- Add the native configuration (Android/iOS)
- Add the Plugin to the Flutter project
Sequence
- Android – Add the native configuration in the Android Studio project
- iOS – Add the native configuration in the Xcode project
- Add the Plugin to your Flutter Project
- Init Method
- Start The PG transaction
- Helper Methods (For Android and iOS)
- Helper Methods (Only Android Specific)
- Get Package Signature for Android
- Get UPI Apps for Android
- Parameters
- Server Side Implementation
- Flutter Sample App
Android
Add the native configuration in the Android Studio project
- Add the below code to ‘repositories’ section of your project level build.gradle file
// Top-level build file where you can add configuration options common to all sub-projects/modules.
allprojects {
repositories {
google()
maven {
url "https://phonepe.mycloudrepo.io/public/repositories/phonepe-intentsdk-android"
}
}
}
iOS
Add the native configuration in the Xcode project
1. In your Info.plist under the iOS Xcode project, create or append a new Array type node LSApplicationQueriesSchemes to append the following values:
<key>LSApplicationQueriesSchemes</key>
<array>
<string>ppemerchantsdkv1</string>
<string>ppemerchantsdkv2</string>
<string>ppemerchantsdkv3</string>
<string>paytmmp</string>
<string>gpay</string>
</array>
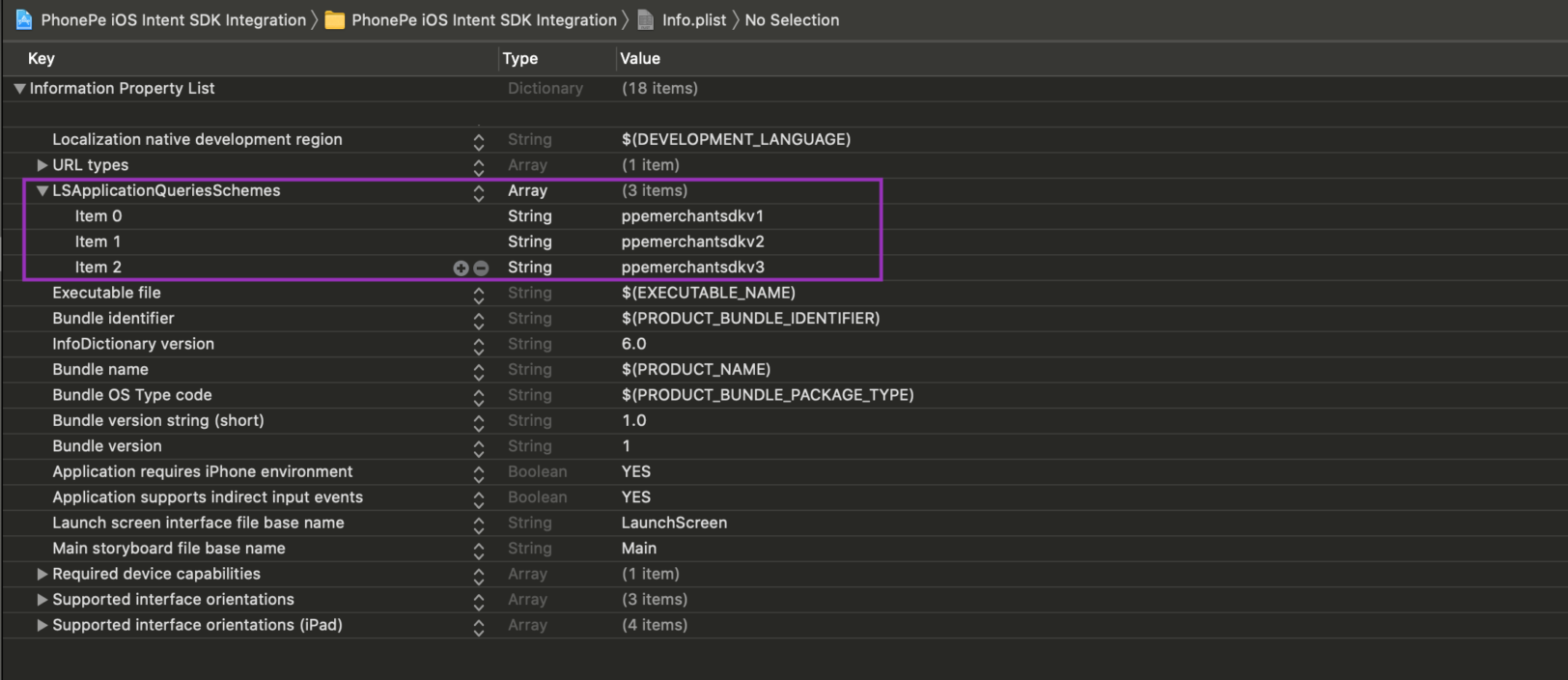
2. Create a URLType for your app (Deeplink), if not already present.
For example, we have used: iOSIntentIntegration. (You can create your own identifier for your app)

URLScheme should match the below conditions
- Only Alphabets (lower and upper case) and numbers are allowed.
- We can allow special characters only like dot and Hyphen
- The name should always start with alphabets.
The schema should be correct to redirect the app otherwise it will not redirect back to the merchant app.
Add the Plugin to your Flutter Project
- Add the dependency in the Flutter project from the command line
flutter pub add phonepe_payment_sdk
- Install the dependency from the command line
flutter pub get
- Import the package in your dart code
import 'package:phonepe_payment_sdk/phonepe_payment_sdk.dart';
- Call the PhonePe methods as below
Init Method
Initialize the init method before starting the transaction.
/**
* This method is used to initiate PhonePe Payment sdk.
* Provide all the information as requested by the method signature.
* Params:
* - environment: This signified the environment required for the payment sdk
* possible values: SANDBOX, PRODUCTION
* if any unknown value is provided, PRODUCTION will be considered as default.
* - merchantId: The merchant id provided by PhonePe at the time of onboarding.
* - appId: It is optional, if you have received it from the PhonePe team, kindly pass it within the method. Otherwise pass it as 'null'.
* - enableLogging: If you want to enabled / visualize sdk log
* - enabled = YES
* - disable = NO
*/
static Future<bool> init(
String environment,
String appId,
String merchantId,
bool enableLogging) async
Example ->
PhonePePaymentSdk.init(environmentValue, appId, merchantId, enableLogging)
.then((val) => {
setState(() {
result = 'PhonePe SDK Initialized - $val';
})
})
.catchError((error) {
handleError(error);
return <dynamic>{};
});
Start The PG transaction
/**
/*
* This method is used to initiate PhonePe B2B PG Flow.
* Provide all the information as requested by the method signature.
* Params:
* - body : The request body for the transaction as per the developer docs.
* - appSchema: @Optional(for Android) Your custom app URL Schemes, as per the developer docs.
* - checkSum: checksum for the particular transaction as per the developer docs.
* - packageName: @Optional(for iOS) in case of android if intent url is expected for specific app.
*
* Return: Will be returning a dictionary / hashMap
* {
* status: String, // string value to provide the status of the transaction
* // possible values: SUCCESS, FAILURE, INTERRUPTED
* error: String // if any error occurs
* }
*/
static Future<Map<dynamic, dynamic>?> startTransaction(String body,
String appSchema, String checksum, String? packageName) async {
var dict =
{
'body': body,
'callbackUrl': appSchema,
'checksum': checksum,
'packageName': packageName
};
Map<dynamic, dynamic>? result =
await _channel.invokeMethod('startTransaction', dict);
return result;
}
Example:
PhonePePaymentSdk.startTransaction(body, callback, checksum, packageName).then((response) => {
setState(() {
if (response != null)
{
String status = response['status'].toString();
String error = response['error'].toString();
if (status == 'SUCCESS')
{
// "Flow Completed - Status: Success!";
}
else {
// "Flow Completed - Status: $status and Error: $error";
}
}
else {
// "Flow Incomplete";
}
})
}).catchError((error) {
// handleError(error)
return <dynamic>{};
});
Helper Methods (Only Android Specific)
Get Package Signature for Android
/**
* This method is called to get package signature while creation of AppId in @Android only.
* Return: String
* Non empty string -> app package signature
* NOTE :- In iOS, it will send null.
*/
static Future<String?> getPackageSignatureForAndroid()
Parameters
Keys | Data Type | Possible Values |
---|---|---|
environment | String | 1. SANDBOX 2. PRODUCTION |
merchantId | String | Add the Merchant ID provided by the Integration Team |
appId | String | It is optional, if you have received it from the PhonePe team, kindly pass it within the method. Otherwise pass it as ‘null’. |
enableLogs | Bool | Show the logs in the console in the SDK 1. true 2. false |
body | String | base64String |
appSchema | String | [Only for iOS]Your App URL Scheme – To return the UI control back to the merchant app. |
checksum | String | Checksum for the particular transaction. Refer |
apiEndPoint | String | The API endpoint for the PG transaction. Refer |
packageName | String | [Only for Android]The Package name of the UPI app selected by the user. |
Server Side Implementation
Step 1. Save the below-assigned value on your server
String apiEndPoint = "/pg/v1/pay";
Step 2. Construct the request body and encode it in Base 64 at your server as per the platform (Android/iOS):
For PG Pay API (Standard Checkout), refer to this Link.
Sample payload
{
"merchantId": "MERCHANTUAT",
"merchantTransactionId": "MT7850590068188104",
"merchantUserId": "MUID123",
"amount": 10000,
"callbackUrl": "https://webhook.site/callback-url",
"mobileNumber": "9999999999",
"paymentInstrument": {
"type": "PAY_PAGE"
}
}
Convert the JSON Payload to Base64 Encoded Payload
The above JSON request payload should be converted to the Base64 Encoded Payload and then the request should be sent in the below format.
Sample Request
{
"request": "ewogICJtZXJjaGFudElkIjogIk1FUkNIQU5UVUFUIiwKICAibWVyY2hhbnRUcmFuc2FjdGlvbklkIjogIk1UNzg1MDU5MDA2ODE4ODEwNCIsCiAgIm1lcmNoYW50VXNlcklkIjogIk1VSUQxMjMiLAogICJhbW91bnQiOiAxMDAwMCwKICAicmVkaXJlY3RVcmwiOiAiaHR0cHM6Ly93ZWJob29rLnNpdGUvcmVkaXJlY3QtdXJsIiwKICAicmVkaXJlY3RNb2RlIjogIlJFRElSRUNUIiwKICAiY2FsbGJhY2tVcmwiOiAiaHR0cHM6Ly93ZWJob29rLnNpdGUvY2FsbGJhY2stdXJsIiwKICAibW9iaWxlTnVtYmVyIjogIjk5OTk5OTk5OTkiLAogICJwYXltZW50SW5zdHJ1bWVudCI6IHsKICAgICJ0eXBlIjogIlBBWV9QQUdFIgogIH0KfQ=="
}
Step 3. Checksum Calculation
Select one of the salts shared with you and note its index. Construct the X-verify at your server as follows:
String checksum = sha256(base64Body + apiEndPoint + salt) + ### + saltIndex;
Step 4. Check the payment status
Once the payment is completed, please call the Check Transaction Status API to validate the response received via the App. You can call the Check Transaction Status at regular intervals to fetch the response from the PhonePe server in case a response is not received in the application even after 10 minutes of initiating the application.
Step 5. Handling Payment Status
The payment status can be Successful, Failed, Pending or any of the codes. For Pending, you should retry until the status changes to Successful or Failed.
Flutter Sample App
Refer to the Sample App to test the Plugin and use it in your Flutter platform – Link